When the simulated slug hits an obstacle straight-on, both sensors fire, which forces an arbitrary choice between turning left and right. The simple Braitenberg circuit doesn’t handled the conflict. And, because of timing and inertial, the slug could start turning right, hit both sensors and switch to a left turn. My prior solution for the problem chose one direction to win, which does work but has problems of its own.
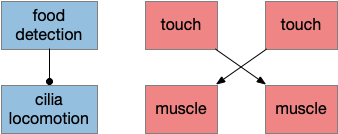
In a natural setting where the animal needs to flee from predators, a predictable route is dangerous. When a zebrafish larva escapes, it chooses left or right randomly. The choice itself doesn’t matter; it’s the unpredictability of a random choice that matters. Unfortunately, the simple circuit doesn’t solve the problem.
Using muscles for timing
One problem is a lack of memory or internal state to make the choice stick. Without memory, and with random choice, the animal chooses again and again, because the previous choice doesn’t lock out the next one. Since both sensors are active, the animal chooses left or right randomly, but 10ms (or one tick) later it notices both sensors active and chooses again. It can’t stick to its decision. Because the animal doesn’t’ have a cortex or any complicated recurrent circuit at all, it doesn’t have working memory in the psychological sense. Besides, a complicated hundreds-of-neurons solution is overkill for this problem, as well as being evolutionarily implausible.
Because it’s a timing problem, and biology is a master of chemistry, either a chemical or a mechanical solution is easier than a circuit solution. A glutamate neuron with a fast receptor (AMPA, for example), is around 5-10ms, which is far too short for physical behavior. I need something in the 500ms range.
The muscle itself is one possible memory source, since the muscles and body motion is what I’m trying to control. Essentially, using physical timing. If a proprioceptive signal from the active muscle could inhibit the opposite muscle, the muscle’s own activity would force a follow-through, avoiding the high-frequency oscillation of random choice.
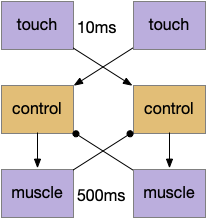
The controller code only fires the muscle if the opposite muscle isn’t firing. There are separate controllers for the left and the right.
fn touch_muscle_feedback_left(mut body: ResMut<Body>) {
if body.is_sensor_left() && ! body.is_muscle_left() {
body.set_muscle_right(1.);
}
}
Using monoamines for attention memory
While muscle proprioception for memory works, it’s limited to near the muscle. Another option in the 500ms range is to use monoamines like dopamine, serotonin, noradrenaline, or to use acetylcholine (ACh), all of which have receptors in the 50-500ms range.
A possible circuit might borrow from the muscle circuit, using dopamine for memory. Instead of the muscles providing negative feedback, a dopamine neuron receives a collateral from muscle activation. The target command interneuron has an inhibitory DA receptor (D2), which prevents it from firing.
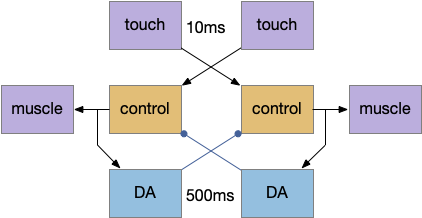
Using dopamine instead of serotonin in this example is mostly arbitrary because many action-related systems use serotonin, and both neurotransmitters have multiple receptor types for both excitation and inhibition. I’m using DA here because of some parallels with the striatum (basal ganglia.)
One of the functions of the striatum/basal ganglia is to lock in choices, like I need here. Once locked-in, the choices need some way of unlocking, such as for a new danger or a new opportunity, or simply to prevent the animal from getting stuck. Here, I’m using the chemical decay to timeout the locked-in choice. In the striatum, ACh neurons and specific thalamic input (specifically T.pf) breaks the current choice and pauses input, allowing for choice switching. This sophisticated attention-breaking system implies that it’s breaking an existing attention-retention system. So, it’s at least conceivable that and attention system like I’m exploring here might have been one of the initial, primitive sources for striatal function.
Code for dopamine attention
fn touch_muscle_dopamine(
mut body: ResMut<Body>,
mut da: Local<DopaminePair>
) {
da.left = (da.left - DopaminePair::DECAY).max(0.);
da.right = (da.right - DopaminePair::DECAY).max(0.);
if body.is_sensor_left() && da.left <= 0. {
body.set_muscle_right(1.);
da.right = if da.right <= 0. { 1. } else { da.right };
}
if body.is_sensor_right() && da.right <= 0. {
body.set_muscle_left(1.);
da.left = if da.left <= 0. { 1. } else { da.left };
}
}
As a coding matter, if the example were any more complicated I’d need to refactor this into something more abstract.
The decay simulates the chemical and receptor signal decay. The two actions are axon collaterals: firing the muscle and activating dopamine. The semi-complicated dopamine assignment simulates a refractory period, where the dopamine can’t fire until its decay is complete. The current code works for a single instance, but it would get annoying for multiple controllers.
The same might be true for evolution. Evolving this system once is plausible, but repeating it for every instance would be difficult, which might explain the evolution of a system like the striatum as a single solution to a repeated problem.
Avoiding positive feedback
I’ve avoided using positive feedback deliberately. First, because I think it’s too large of a step for evolution to create for such a simple system. Second, because positive feedback can get stuck without the addition of a reset signal, which again is too much to ask of evolution. Both the muscle timing and chemical timing solutions are capabilities already available, are more robust, and are simpler solutions.
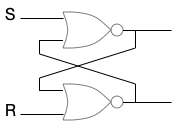
The set-reset latch above shows some of the complexities. The latch needs both a set and a reset signal. Unless there’s some mechanism to reset the latch, it’s not practical. But adding a reset is another step for evolution, and to add both the feedback and a reset simultaneously is a difficult ask.
Second, the electrical latch works because it can maintain two constant digital values. Either is a stable state. A neuron only has one stable state and sends signals with pulses, and early neurons were likely exceptionally noisy. So, there’s a good chance for an ON signal to get lost just because of noise. Or, if the gain is exceptionally high to keep the ON, then noise might generate a spurious signal.
Now, feedback signals do exist in the brain — the hippocampus CA3 is an important example — but those have a far greater complexity than the simple slug I’m considering here. For example, there’s a sophisticated entire dual clocking system (theta and gamma) and ACh reset system in CA3 [Hasselmo 2006].
References
Hasselmo ME. The role of acetylcholine in learning and memory. Curr Opin Neurobiol. 2006 Dec;16(6):710-5. doi: 10.1016/j.conb.2006.09.002. Epub 2006 Sep 29. PMID: 17011181; PMCID: PMC2659740.